Recently, I received a message from our Operations center that they had seen 20+ Continuous test accounts on one of our Session Hosts. Fortunately, they had previously drained users from that server, so this had not impacted our customer base. But, it was a concern, since this would have put the host very close to its capacity.
For some context, we are hosting applications using MS Remote Desktop Services. We have a continuous test defined for each host.
This happened in our smallest datacenter where we have only 118 continuous tests running. But, I didn’t want to click into 118 tests to check the Target host. So, I knocked up a quick Powershell script to pull the host for each test into a .CSV file.
I thought I would share this in case anyone has a similar need and can benefit from looking at a script for inspiration/example.
As I stated in my previous post about writing a script for Capacity Planning, https://ssun-blog.net/index.php/2023/10/30/capacity-planning/ , I used the LoginEnterpriseFunctions.ps1 as the foundation for my scripts, although, in this case, I reused a script that we have that enables/disables all of our tests for maintenance windows.
This is a pretty simple script.
Header
The header of the script is where I define the variables that will be used later in the script.
Add-Type -AssemblyName PresentationFramework
$global:fqdn = "<appliance URL>"
$global:token = '<appliance API Token>'
$global:response = " "
$timestamp = Get-Date -Format o | ForEach-Object { $_ -replace ":", "." }
$report=".\data\TestTargets.txt"
The $global:fqdn and $global:Token are what will used by the RestAPI call to connect with the appliance.
I will save the output of the script to the file defined in the $report object.
Get-Test Function
This is pretty much taken right out of the LoginEnterpriseFunctions script. The are two modifications:
- I changed the Uri Parameter to use the v7-preview API instead of the v4 API.
- I changed the include parameter to “connectionResources” to target the information I was looking for.
function Get-Tests {
# Param (
# [Parameter(Mandatory)] [ValidateSet('name', 'connector', 'description')] [string] $orderBy,
# [Parameter(Mandatory)] [ValidateSet('continuousTest', 'loadTest', 'applicationTest')] [string] $testType,
# [Parameter(Mandatory)] [ValidateSet('ascending', 'descending')] [string] $direction,
# [Parameter(Mandatory)] [ValidateSet('environment', 'workload', 'thresholds', 'all')] [string] $include,
# [Parameter(Mandatory)] [string]$count
# )
# this is only required for older version of PowerShell/.NET
[Net.ServicePointManager]::SecurityProtocol = [Net.SecurityProtocolType]::Tls12 -bor [Net.SecurityProtocolType]::Tls11
# WARNING: ignoring SSL/TLS certificate errors is a security risk
[System.Net.ServicePointManager]::ServerCertificateValidationCallback = [SSLHandler]::GetSSLHandler()
$Header = @{
"Accept" = "application/json"
"Authorization" = "Bearer $global:token"
}
$Body = @{
# testType = $testType
orderBy = "name"
direction = "asc"
count = 1000
include = "connectionResources"
}
$Parameters = @{
Uri = 'https://' + $global:fqdn + '/publicApi/v7-preview/tests'
Headers = $Header
Method = 'GET'
body = $Body
ContentType = 'application/json'
}
$Response = Invoke-RestMethod @Parameters
$Response.items
}
Logic
The rest of the script is the logic to pull the name of the test and the target host for the RDP connections.
I first write the header row to the CSV file, using the -Force parameter to overwrite any existing file. NOTE: for other scripts, I will incorporate the $timestamp defined in the header in the file name so that I can keep historical records.
Next, I call the Get-Tests function and place that in the $tests array object.
The ForEach loop iterates through each record in the array and places the current record into the $test object.
Then, using the Out-File cmdlet with the -Append parameter, I write out the Name of the test, a comma and then the Target name, which is stored in the subarrays of $test –> connectionResources –> hostlist –> endpoint.
To help you visualize the structure, here is a screenshot of the $test object in JSON Buddy.
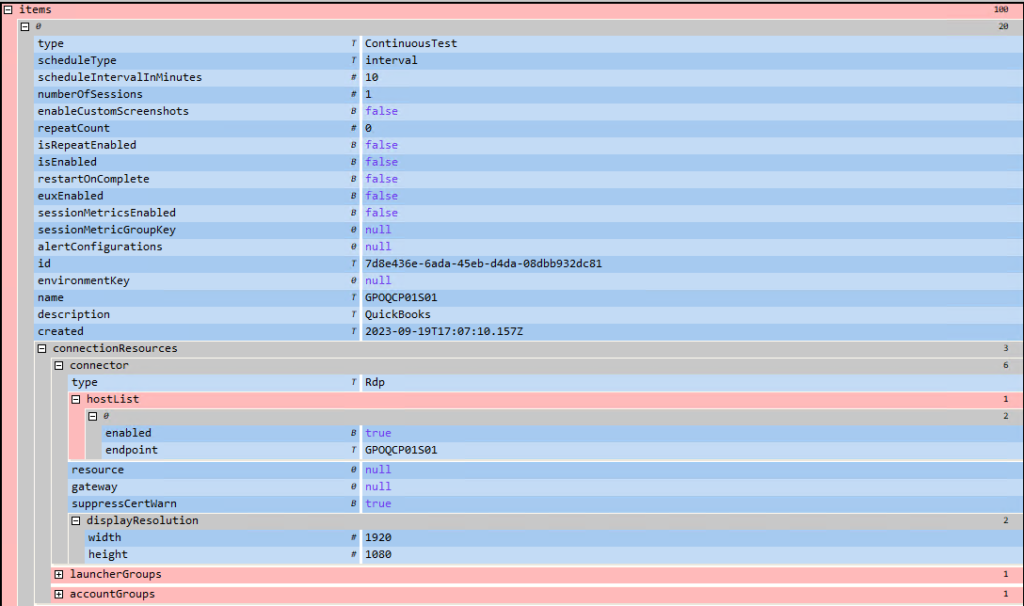
Conclusion
Using this script, I was able to identify that 15 of our tests were configured to use the same Session Host. As it was only 15 tests that need to be corrected, I manually changed them, but otherwise I would have written a script to do that! 🙂
Script
Here is the script in its entirety